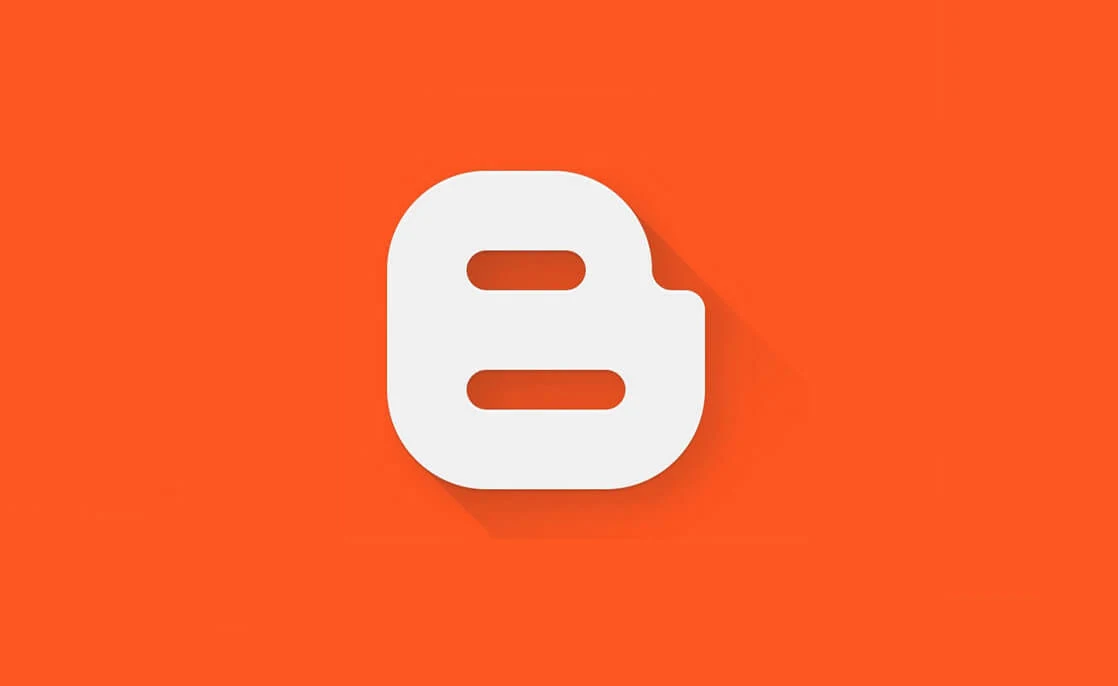
How To Start a Blogger Blog: blogspot
Understanding React Hooks: A Comprehensive Guide
React Hooks have revolutionized the way we write React components. In this comprehensive guide, we'll explore what Hooks are, why they were introduced, and how to use them effectively in your React applications.
Introduction to React Hooks
React Hooks were introduced in React 16.8 as a way to use state and other React features without writing a class. They allow you to "hook into" React state and lifecycle features from function components.
Types of React Hooks
useState
The useState
hook allows you to add state to your functional components. Here's a simple example:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
useEffect
The useEffect
hook lets you perform side effects in function components. It serves the same purpose as componentDidMount
, componentDidUpdate
, and componentWillUnmount
in React classes.
import React, { useState, useEffect } from 'react';
function Example() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
});
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
Best Practices for Using Hooks
- Only call Hooks at the top level of your React function.
- Only call Hooks from React function components or custom Hooks.
- Use the ESLint plugin
eslint-plugin-react-hooks
to enforce these rules.
Conclusion
React Hooks provide a more direct API to the React concepts you already know: props, state, context, refs, and lifecycle. They don't fundamentally change how React works, but they do provide a more ergonomic way to reuse stateful logic between components.
By mastering Hooks, you can write more concise and easier-to-understand React components. Happy coding!